介绍
打包出来的 apk ipa 安装在同一部手机上经常无法分辨,如果能在图标上增加一些文字信息,会非常方便。
参考
需求
- 跨平台,需要同时支持 Windows 与 macOS。上面列出了一些参考链接,但是都是处理 iOS 平台的,因此大部分都使用了 shell 脚本。
- 依赖少,尽量减少依赖,降低配置环境成本。
根据实际需求,使用 Python + Imagemagick 方式实现,不再使用 Python 的 ImageMagick 绑定库,如 Wand — Wand 0.5.5 之类的。
其次,为了减少依赖,需要简化需求,只处理一张图片,处理多张图片就必然需要读取图片大小等信息,使用 Python 调用命令并不方便获得此信息。
环境
- Windows 7
- macOS 10.14.5
- Python 3.7.3
- ImageMagick 7.0.8
准备
Windows
建议安装官方推荐的版本:ImageMagick-7.0.8-53-Q16-x64-dll.exe
注意在安装的过程中一定要勾选安装 install legacy tools (such as convert)
,这样才会安装 convert
命令。
macOS
使用 HomeBrew 安装即可
1
|
brew install imagemagick
|
实现
以下代码功能是将图标添加水印
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
|
#!/usr/bin/env python
# -*- coding: UTF-8 -*-
import datetime
import os
import platform
import tempfile
def main():
change_app_icon('AppIcon.png', 280, 'Alpha', 'v0.1.0')
def change_app_icon(app_icon_path, image_size, channel, version):
if not os.path.exists(app_icon_path):
print(f'"{app_icon_path} didn\'t exist')
return
if platform.system() == 'Windows':
convert_path = 'C:\\Program Files\\ImageMagick-7.0.8-Q16\\convert.exe'
composite_path = 'C:\\Program Files\\ImageMagick-7.0.8-Q16\\composite.exe'
else:
convert_path = '/usr/local/bin/convert'
composite_path = '/usr/local/bin/composite'
if not os.path.exists(convert_path):
print('Imagemagick didn\'t install. Please install Imagemagick first.')
return
crop_height = image_size / 2
font_size = image_size * 8 / 58
date = datetime.datetime.now().strftime("%Y%m%d")
if platform.system() == 'Windows':
caption = f'{channel}\\n{version}\\n{date}'
else:
caption = f'"{channel}\\n{version}\\n{date}"'
tempdir = tempfile.gettempdir()
blurred_icon = os.path.join(tempdir, 'app-icon-blurred.png')
cropped_blurred_icon = os.path.join(tempdir, 'app-icon-cropped-blurred.png')
label_icon = os.path.join(tempdir, 'app-icon-label.png')
os.system(f'"{convert_path}" {app_icon_path} -blur 8x8 {blurred_icon}')
os.system(f'"{convert_path}" {blurred_icon} -crop {image_size}x{crop_height}+0+{image_size-crop_height} '
f'{cropped_blurred_icon}')
os.system(f'"{convert_path}" -background none -fill white -pointsize {font_size} -gravity center '
f'caption:{caption} {cropped_blurred_icon} +swap -composite {label_icon}')
os.system(f'"{composite_path}" -geometry +0+{image_size-crop_height} {label_icon} '
f'{app_icon_path} {app_icon_path}')
if __name__ == '__main__':
main()
|
以上代码分别在 Windows、macOS 平台上测试通过。
注意:图标路径不要包含空格,否则会解析错误。
结果
添加前
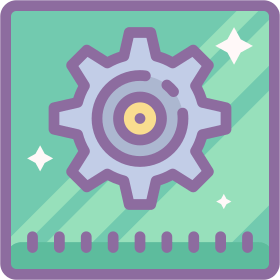
添加后
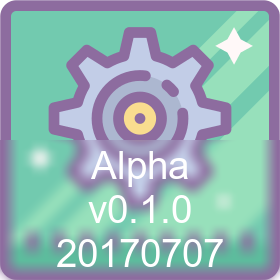